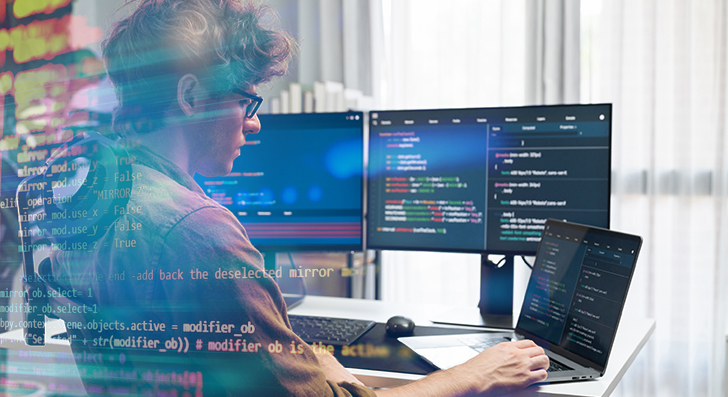
Scalability signifies your software can cope with progress—a lot more customers, far more info, and even more visitors—without breaking. To be a developer, constructing with scalability in mind will save time and anxiety later on. Right here’s a transparent and useful guide to assist you to start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability isn't really one thing you bolt on afterwards—it should be section of the plan from the start. Many apps fail whenever they expand fast due to the fact the original layout can’t handle the extra load. To be a developer, you should Imagine early regarding how your system will behave under pressure.
Get started by developing your architecture being flexible. Keep away from monolithic codebases where every little thing is tightly related. Alternatively, use modular structure or microservices. These patterns split your application into smaller, independent areas. Each module or support can scale By itself with out impacting The full procedure.
Also, consider your database from working day a person. Will it need to deal with one million users or perhaps a hundred? Select the suitable style—relational or NoSQL—according to how your info will increase. System for sharding, indexing, and backups early, even if you don’t want them nevertheless.
A different vital point is to avoid hardcoding assumptions. Don’t create code that only operates beneath recent conditions. Think about what would come about In case your user base doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design styles that guidance scaling, like message queues or event-pushed units. These assistance your application cope with additional requests devoid of finding overloaded.
Any time you Establish with scalability in your mind, you are not just getting ready for achievement—you are decreasing future problems. A very well-prepared process is simpler to maintain, adapt, and develop. It’s better to arrange early than to rebuild later on.
Use the correct Database
Deciding on the suitable database can be a crucial A part of building scalable apps. Not all databases are developed exactly the same, and utilizing the Mistaken one can gradual you down or simply lead to failures as your app grows.
Get started by knowledge your info. Can it be hugely structured, like rows inside a desk? If Indeed, a relational databases like PostgreSQL or MySQL is a superb in shape. They are potent with associations, transactions, and regularity. Additionally they support scaling tactics like study replicas, indexing, and partitioning to take care of much more visitors and facts.
Should your details is much more adaptable—like user action logs, product catalogs, or paperwork—take into consideration a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at managing large volumes of unstructured or semi-structured knowledge and will scale horizontally a lot more conveniently.
Also, think about your examine and write designs. Are you presently undertaking lots of reads with fewer writes? Use caching and browse replicas. Are you presently handling a large generate load? Look into databases that will cope with high compose throughput, or simply occasion-based mostly facts storage systems like Apache Kafka (for short-term info streams).
It’s also clever to Imagine ahead. You may not require Innovative scaling features now, but choosing a database that supports them implies you gained’t need to have to modify afterwards.
Use indexing to hurry up queries. Stay clear of unnecessary joins. Normalize or denormalize your data dependant upon your entry styles. And generally observe databases general performance when you mature.
In short, the right databases depends on your app’s construction, speed needs, and how you expect it to grow. Take time to select sensibly—it’ll help save many difficulties later on.
Enhance Code and Queries
Rapidly code is vital to scalability. As your app grows, every compact hold off provides up. Badly created code or unoptimized queries can decelerate general performance and overload your process. That’s why it’s important to Establish successful logic from the start.
Begin by writing cleanse, basic code. Stay away from repeating logic and remove just about anything unneeded. Don’t choose the most complex solution if a straightforward one particular functions. Keep the features short, concentrated, and simple to check. Use profiling equipment to locate bottlenecks—sites where by your code normally takes as well extensive to run or uses an excessive amount memory.
Subsequent, evaluate your database queries. These normally sluggish matters down a lot more than the code itself. Be certain Each and every question only asks for the data you truly require. Prevent Choose *, which fetches anything, and rather pick out particular fields. Use indexes to hurry up lookups. And avoid accomplishing too many joins, Specially throughout big tables.
When you notice precisely the same details getting asked for many times, use caching. Shop the final results temporarily employing applications like Redis or Memcached so that you don’t really need to repeat highly-priced operations.
Also, batch your database operations if you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and helps make your app more effective.
Remember to examination with substantial datasets. Code and queries that work good with 100 information may possibly crash if they have to take care of one million.
In short, scalable applications are fast apps. Maintain your code restricted, your queries lean, and use caching when essential. These techniques enable your software continue to be sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to manage additional users and much more site visitors. If every little thing goes by means of a single server, it is going to speedily become a bottleneck. That’s in which load balancing and caching come in. These two tools assistance keep the application speedy, secure, and scalable.
Load balancing spreads incoming targeted traffic across several servers. Rather than 1 server doing many of the do the job, the load balancer routes people to diverse servers depending on availability. This implies no one server will get overloaded. If just one server goes down, the load balancer can send out traffic to the others. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to arrange.
Caching is about storing knowledge temporarily so it might be reused speedily. When customers ask for precisely the same info once more—like an item webpage or perhaps a profile—you don’t really need to fetch it through the database anytime. You'll be able to provide it from your cache.
There are two widespread kinds of caching:
one. Server-side caching (like Redis or Memcached) outlets facts in memory for quick obtain.
2. Customer-side caching (like browser caching or CDN caching) merchants static files near to the person.
Caching decreases databases load, improves pace, and makes your application more economical.
Use caching for things that don’t transform frequently. And generally make sure your cache is up-to-date when details does modify.
To put it briefly, load balancing and caching are easy but highly effective tools. Collectively, they assist your application deal with additional consumers, keep fast, and Recuperate from challenges. If you propose to develop, you may need both of those.
Use Cloud and Container Resources
To create scalable apps, you would like resources that allow your application improve easily. That’s exactly where cloud platforms and containers are available. They give you flexibility, minimize setup time, and make scaling Substantially smoother.
Cloud platforms like Amazon Website Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to rent servers and providers as you may need them. You don’t should invest in components or guess future capacity. When traffic will increase, you may insert additional means with just some clicks or mechanically applying vehicle-scaling. When targeted visitors drops, you could scale down read more to economize.
These platforms also present expert services like managed databases, storage, load balancing, and protection instruments. It is possible to target constructing your application as an alternative to controlling infrastructure.
Containers are Yet another crucial Instrument. A container packages your application and almost everything it has to run—code, libraries, configurations—into just one unit. This makes it easy to maneuver your app in between environments, from a laptop computer to your cloud, with no surprises. Docker is the most well-liked tool for this.
Once your app uses various containers, instruments like Kubernetes enable you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single part within your app crashes, it restarts it automatically.
Containers also help it become simple to different areas of your app into services. You may update or scale components independently, which happens to be great for performance and dependability.
In short, working with cloud and container resources usually means you'll be able to scale speedy, deploy very easily, and Get better swiftly when complications take place. If you want your app to improve with no limits, start off applying these resources early. They help save time, decrease chance, and help you remain centered on setting up, not fixing.
Keep an eye on Everything
Should you don’t watch your software, you won’t know when items go Erroneous. Checking assists you see how your application is carrying out, place difficulties early, and make better decisions as your app grows. It’s a essential Element of building scalable techniques.
Start off by monitoring primary metrics like CPU use, memory, disk space, and response time. These let you know how your servers and providers are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you collect and visualize this information.
Don’t just check your servers—observe your application too. Keep an eye on how long it takes for customers to load pages, how often errors occur, and in which they take place. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Setup alerts for essential issues. For instance, In case your response time goes higher than a Restrict or maybe a service goes down, you should get notified straight away. This allows you deal with troubles rapidly, usually prior to customers even notice.
Checking can be beneficial whenever you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, you may roll it back again before it results in true injury.
As your application grows, targeted visitors and facts boost. Without checking, you’ll skip indications of difficulties till it’s far too late. But with the correct tools in position, you stay on top of things.
In brief, checking assists you keep the app trusted and scalable. It’s not nearly recognizing failures—it’s about knowing your procedure and ensuring it really works nicely, even stressed.
Final Views
Scalability isn’t just for significant organizations. Even compact apps will need a strong foundation. By building very carefully, optimizing sensibly, and using the appropriate tools, you'll be able to Establish apps that increase effortlessly without having breaking stressed. Start tiny, Imagine large, and Create good.